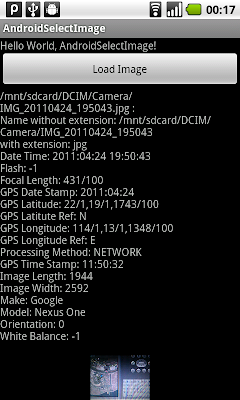
A new class MyExif is implemented to handle the operation on the JPG file, it's used to read EXIF. (May be more functions will be added later)
Note that it's target for API Level 8, Android 2.2, other version will provide more or less TAG of EXIF.
MyExif.java
package com.exercise.AndroidSelectImage;
import java.io.File;
import java.io.IOException;
import android.app.Activity;
import android.database.Cursor;
import android.media.ExifInterface;
import android.net.Uri;
import android.provider.MediaStore;
public class MyExif {
private File exifFile; //It's the file passed from constructor
private String exifFilePath; //file in Real Path format
private Activity parentActivity; //Parent Activity
private String exifFilePath_withoutext;
private String ext;
private ExifInterface exifInterface;
private Boolean exifValid = false;;
//Exif TAG
//for API Level 8, Android 2.2
private String exif_DATETIME = "";
private String exif_FLASH = "";
private String exif_FOCAL_LENGTH = "";
private String exif_GPS_DATESTAMP = "";
private String exif_GPS_LATITUDE = "";
private String exif_GPS_LATITUDE_REF = "";
private String exif_GPS_LONGITUDE = "";
private String exif_GPS_LONGITUDE_REF = "";
private String exif_GPS_PROCESSING_METHOD = "";
private String exif_GPS_TIMESTAMP = "";
private String exif_IMAGE_LENGTH = "";
private String exif_IMAGE_WIDTH = "";
private String exif_MAKE = "";
private String exif_MODEL = "";
private String exif_ORIENTATION = "";
private String exif_WHITE_BALANCE = "";
//Constructor from path
MyExif(String fileString, Activity parent){
exifFile = new File(fileString);
parentActivity = parent;
exifFilePath = fileString;
PrepareExif();
}
//Constructor from URI
MyExif(Uri fileUri, Activity parent){
exifFile = new File(fileUri.toString());
parentActivity = parent;
exifFilePath = getRealPathFromURI(fileUri);
PrepareExif();
}
private void PrepareExif(){
int dotposition= exifFilePath.lastIndexOf(".");
exifFilePath_withoutext = exifFilePath.substring(0,dotposition);
ext = exifFilePath.substring(dotposition + 1, exifFilePath.length());
if (ext.equalsIgnoreCase("jpg")){
try {
exifInterface = new ExifInterface(exifFilePath);
ReadExifTag();
exifValid = true;
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
private void ReadExifTag(){
exif_DATETIME = exifInterface.getAttribute(ExifInterface.TAG_DATETIME);
exif_FLASH = exifInterface.getAttribute(ExifInterface.TAG_FLASH);
exif_FOCAL_LENGTH = exifInterface.getAttribute(ExifInterface.TAG_FOCAL_LENGTH);
exif_GPS_DATESTAMP = exifInterface.getAttribute(ExifInterface.TAG_GPS_DATESTAMP);
exif_GPS_LATITUDE = exifInterface.getAttribute(ExifInterface.TAG_GPS_LATITUDE);
exif_GPS_LATITUDE_REF = exifInterface.getAttribute(ExifInterface.TAG_GPS_LATITUDE_REF);
exif_GPS_LONGITUDE = exifInterface.getAttribute(ExifInterface.TAG_GPS_LONGITUDE);
exif_GPS_LONGITUDE_REF = exifInterface.getAttribute(ExifInterface.TAG_GPS_LONGITUDE_REF);
exif_GPS_PROCESSING_METHOD = exifInterface.getAttribute(ExifInterface.TAG_GPS_PROCESSING_METHOD);
exif_GPS_TIMESTAMP = exifInterface.getAttribute(ExifInterface.TAG_GPS_TIMESTAMP);
exif_IMAGE_LENGTH = exifInterface.getAttribute(ExifInterface.TAG_IMAGE_LENGTH);
exif_IMAGE_WIDTH = exifInterface.getAttribute(ExifInterface.TAG_IMAGE_WIDTH);
exif_MAKE = exifInterface.getAttribute(ExifInterface.TAG_MAKE);
exif_MODEL = exifInterface.getAttribute(ExifInterface.TAG_MODEL);
exif_ORIENTATION = exifInterface.getAttribute(ExifInterface.TAG_ORIENTATION);
exif_WHITE_BALANCE = exifInterface.getAttribute(ExifInterface.TAG_WHITE_BALANCE);
}
private String getRealPathFromURI(Uri contentUri) {
String[] proj = { MediaStore.Images.Media.DATA };
Cursor cursor = parentActivity.managedQuery(contentUri, proj, null, null, null);
int column_index = cursor.getColumnIndexOrThrow(MediaStore.Images.Media.DATA);
cursor.moveToFirst();
return cursor.getString(column_index);
}
public String getSummary(){
if(!exifValid){
return ("Invalide EXIF!");
}else{
return( exifFilePath + " : \n" +
"Name without extension: " + exifFilePath_withoutext + "\n" +
"with extension: " + ext + "\n" +
"Date Time: " + exif_DATETIME + "\n" +
"Flash: " + exif_FLASH + "\n" +
"Focal Length: " + exif_FOCAL_LENGTH + "\n" +
"GPS Date Stamp: " + exif_GPS_DATESTAMP + "\n" +
"GPS Latitude: " + exif_GPS_LATITUDE + "\n" +
"GPS Latitute Ref: " + exif_GPS_LATITUDE_REF + "\n" +
"GPS Longitude: " + exif_GPS_LONGITUDE + "\n" +
"GPS Longitude Ref: " + exif_GPS_LONGITUDE_REF + "\n" +
"Processing Method: " + exif_GPS_PROCESSING_METHOD + "\n" +
"GPS Time Stamp: " + exif_GPS_TIMESTAMP + "\n" +
"Image Length: " + exif_IMAGE_LENGTH + "\n" +
"Image Width: " + exif_IMAGE_WIDTH + "\n" +
"Make: " + exif_MAKE + "\n" +
"Model: " + exif_MODEL + "\n" +
"Orientation: " + exif_ORIENTATION + "\n" +
"White Balance: " + exif_WHITE_BALANCE + "\n");
}
}
}
AndroidSelectImage.java
package com.exercise.AndroidSelectImage;
import java.io.File;
import java.io.FileNotFoundException;
import android.app.Activity;
import android.content.Intent;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.net.Uri;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.TextView;
public class AndroidSelectImage extends Activity {
TextView textTargetUri;
ImageView targetImage;
File targetFile;
String exifAttribute;
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
Button buttonLoadImage = (Button)findViewById(R.id.loadimage);
textTargetUri = (TextView)findViewById(R.id.targeturi);
targetImage = (ImageView)findViewById(R.id.targetimage);
buttonLoadImage.setOnClickListener(new Button.OnClickListener(){
@Override
public void onClick(View arg0) {
// TODO Auto-generated method stub
Intent intent = new Intent(Intent.ACTION_PICK,
android.provider.MediaStore.Images.Media.EXTERNAL_CONTENT_URI);
startActivityForResult(intent, 0);
}});
}
@Override
protected void onActivityResult(int requestCode,
int resultCode, Intent data) {
// TODO Auto-generated method stub
super.onActivityResult(requestCode, resultCode, data);
if (resultCode == RESULT_OK){
Uri targetUri = data.getData();
Bitmap bitmap;
try {
bitmap = BitmapFactory.decodeStream(getContentResolver()
.openInputStream(targetUri));
targetImage.setImageBitmap(bitmap);
} catch (FileNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
MyExif myExif = new MyExif(targetUri, this);
textTargetUri.setText(myExif.getSummary());
}
}
}
main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/hello"
/>
<Button
android:id="@+id/loadimage"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Load Image"
/>
<TextView
android:id="@+id/targeturi"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
<ImageView
android:id="@+id/targetimage"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
/>
</LinearLayout>
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.exercise.AndroidSelectImage"
android:versionCode="1"
android:versionName="1.0">
<application android:icon="@drawable/icon" android:label="@string/app_name">
<activity android:name=".AndroidSelectImage"
android:label="@string/app_name">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
<uses-sdk android:minSdkVersion="8" />
</manifest>

Related article:
- Update GPS TAG, using ExifInterface.setAttribute() and exifInterface.saveAttributes()
Thank you for reading this article Read EXIF of JPG file With URL http://x-tutorials.blogspot.com/2011/04/read-exif-of-jpg-file.html. Also a time to read the other articles.
0 comments:
Write your comment for this article Read EXIF of JPG file above!